728x90
반응형
스프링부트 블로그 만들기는
mvc패턴에서 view를 제외한 model과 controller 부분만 포스팅할 예정이니,
view 구현을 원하시는 분들은 프론트엔드 기초 강의를 들으시기를 권합니다.
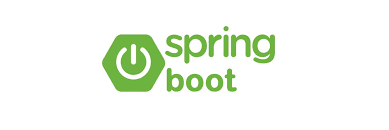
1. ExceptionHandler 구현
package shop.mtcoding.blog.handler;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
import shop.mtcoding.blog.dto.ResponseDto;
import shop.mtcoding.blog.handler.exception.CustomApiException;
import shop.mtcoding.blog.handler.exception.CustomException;
import shop.mtcoding.blog.util.Script;
@RestControllerAdvice
public class CustomExceptionHandler {
@ExceptionHandler(CustomException.class)
public ResponseEntity<?> customException(CustomException e) {
return new ResponseEntity<>(Script.back(e.getMessage()), e.getStatus());
}
@ExceptionHandler(CustomApiException.class)
public ResponseEntity<?> customApiException(CustomApiException e) {
return new ResponseEntity<>(new ResponseDto<>(-1, e.getMessage(), null), e.getStatus());
}
}
@ControllerAdvice와 @RestControllerAdvice
@ControllerAdvice는 단순히 예외만 처리할 때 사용.
@RestControllerAdvice는 응답으로 객체를 리턴할 때 사용.
@ExceptionHandler
Controller계층에서 발생하는 에러를 잡아서 메서드로 처리해주는 라이브러리.
(Service, Repository에서 발생하는 에러는 제외)
2. CustomException 구현
import org.springframework.http.HttpStatus;
import lombok.Getter;
@Getter
public class CustomException extends RuntimeException {
private HttpStatus status;
public CustomException(String msg, HttpStatus status) {
super(msg);
this.status = status;
}
public CustomException(String msg) {
this(msg, HttpStatus.BAD_REQUEST);
// super(msg);
// this.status = HttpStatus.BAD_REQUEST;
}
}
CustomException은 응답으로, 자바스크립트 코드와 상태코드를 리턴합니다.
3. CustomApiException 구현
import org.springframework.http.HttpStatus;
import lombok.Getter;
@Getter
public class CustomApiException extends RuntimeException {
private HttpStatus status;
public CustomApiException(String msg, HttpStatus status) {
super(msg);
this.status = status;
}
public CustomApiException(String msg) {
this(msg, HttpStatus.BAD_REQUEST);
// super(msg);
// this.status = HttpStatus.BAD_REQUEST;
}
}
CustomApiException은 자바 object와 상태코드를 리턴합니다.
CustomException은 Ajax 통신을 할 때 사용이 불가능합니다.
그 이유는 Ajax 통신을 하게 되면 제어권은 자바스크립트가 가지고 있는데,
fail시, 자바스크립트 코드를 받게 되는데,
이때 자바스크립트는 받은 코드를 자바스크립트 object로 파싱하려고 동작합니다.
그래서 자바스크립트 코드를 단지 문자열 데이터로 인식하고, 문자열을 리턴하게 됩니다.
그에 반해, CustomApiException으로 메세지를 받으면,
그것을 자바스크립트 object로 파싱해서 정상적인 결과값을 리턴하게 됩니다.
이렇게 Exception 처리까지 알아보았습니다.
다음 편에는 로그인을 포스팅하겠습니다.
728x90
반응형
'Projects > 스프링부트 블로그 프로젝트' 카테고리의 다른 글
[Spring Boot]스프링부트 블로그 만들기 - 회원가입 구현 (0) | 2023.02.15 |
---|---|
[Spring Boot]스프링부트 블로그 만들기 - 초기 세팅 (0) | 2023.02.15 |